Start using Sledge and Hydrogen
Prior using all Sledge's widgets, follow the steps below to start integrating Sledge into your Hydrogen storefront.
Quickstart
Install Sledge Core
First, you need to install the core of sledge package, run the following command:
npm i @sledge-app/core
Setup the SledgeProvider
Once you've installed the core, you can start using it in your Hydrogen project.
import { type LinksFunction } from "@shopify/remix-oxygen";
import { SledgeProvider } from "@sledge-app/core";
import sledgeCss from '@sledge-app/core/style.css';
export const links: LinksFunction = () => {
return [
...
{ rel: "stylesheet", href: sledgeCss },
...
];
};
export default function App() {
return (
<html lang="en">
<head>
<Meta />
<Links />
</head>
<body>
<SledgeProvider
config={{
userId: "",
userEmail: "",
userFullname: "",
domain: ""
}}
>
{/* Routes, Pages, etc */}
</SledgeProvider>
</body>
</html>
);
}
SledgeProvider
Parameters
Let's look on list of all available parameters:
Parameter | Type | Description |
---|---|---|
userId | string | Fill with your own user logined data, |
userEmail | string | Fill with your own user logined data, |
userFullname | string | Fill with your own user logined data, |
domain | string (required) | Fill with your shop's |
Install one of the Sledge's widget
We're going to try implement product filters widget so install @sledge-app/react-instant-search
package first :
npm i @sledge-app/react-instant-search
Implement Widget
Create a new directory for your components (if it doesn't already exist).
For example, you can create a directory named components
and create a file named SledgeProductFilterWidget.tsx
.
- SledgeProductFilterWidget.tsx
import { ProductFilterWidget } from "@sledge-app/react-instant-search";
import { parseGid } from "@shopify/hydrogen";
export const SledgeProductFilterWidget = ({
collection,
}: {
collection: { id: string; name: string; };
}) => (
<div key={collection?.id}>
<ProductFilterWidget
query={{
keyword: "q",
}}
params={{
collectionId: Number(parseGid(collection?.id).id),
collectionName: collection?.name,
}}
/>
</div>
);
ProductFilterWidget
Parameters
Let's look on list of all available parameters:
Parameter | Type | Description |
---|---|---|
keyword | string | Fill up your unique |
collectionId | string (required) | Fill with your own collection id, |
collectionName | string (required) | Fill with your own collection name, |
Create collections route
Create a new route for your collections page named collections.$handle.tsx
- collections.$handle.tsx
In collections.$handle.tsx
, import your SledgeProductFilterWidget.tsx
component and use it to render ProductFilterWidget
import { SledgeProductFilterWidget } from "~/components/SledgeProductFilterWidget";
import { useLoaderData } from "@remix-run/react";
import { defer, LoaderArgs } from "@shopify/remix-oxygen";
export async function loader({ params, context }: LoaderArgs) {
const { handle } = params;
const { collection } = await context.storefront.query(COLLECTION_QUERY, {
variables: {
handle,
},
});
return defer({
collection,
});
}
export default function Collections() {
const { collection } = useLoaderData<typeof loader>();
return <SledgeProductFilterWidget collection={collection} />;
}
const COLLECTION_QUERY = `#graphql
query CollectionDetails($handle: String!) {
collection(handle: $handle) {
id
title
description
handle
}
}
`;
Add a content security policy
On store site, open browser devtools (opens in a new tab) › Network
tab.
If store success to fetch sledge api you can skip this step.

...
const {nonce, header, NonceProvider} = createContentSecurityPolicy({
connectSrc: [
"'self'",
'http://api.sledge-app.com',
'https://api.sledge-app.com',
'*',
],
defaultSrc: [
'https://shopify.com',
'http://api.sledge-app.com',
'https://api.sledge-app.com',
'data:',
'blob:',
'*',
],
imgSrc: ['*', 'https://api.sledge-app.com', 'data:'],
});
...
If
createContentSecurityPolicy
does not exist on your Hydrogen app, follow this shopify docs (opens in a new tab) for adding a content security policy.
Widget completely running
Sledge's product filters widget is now ready to be used.
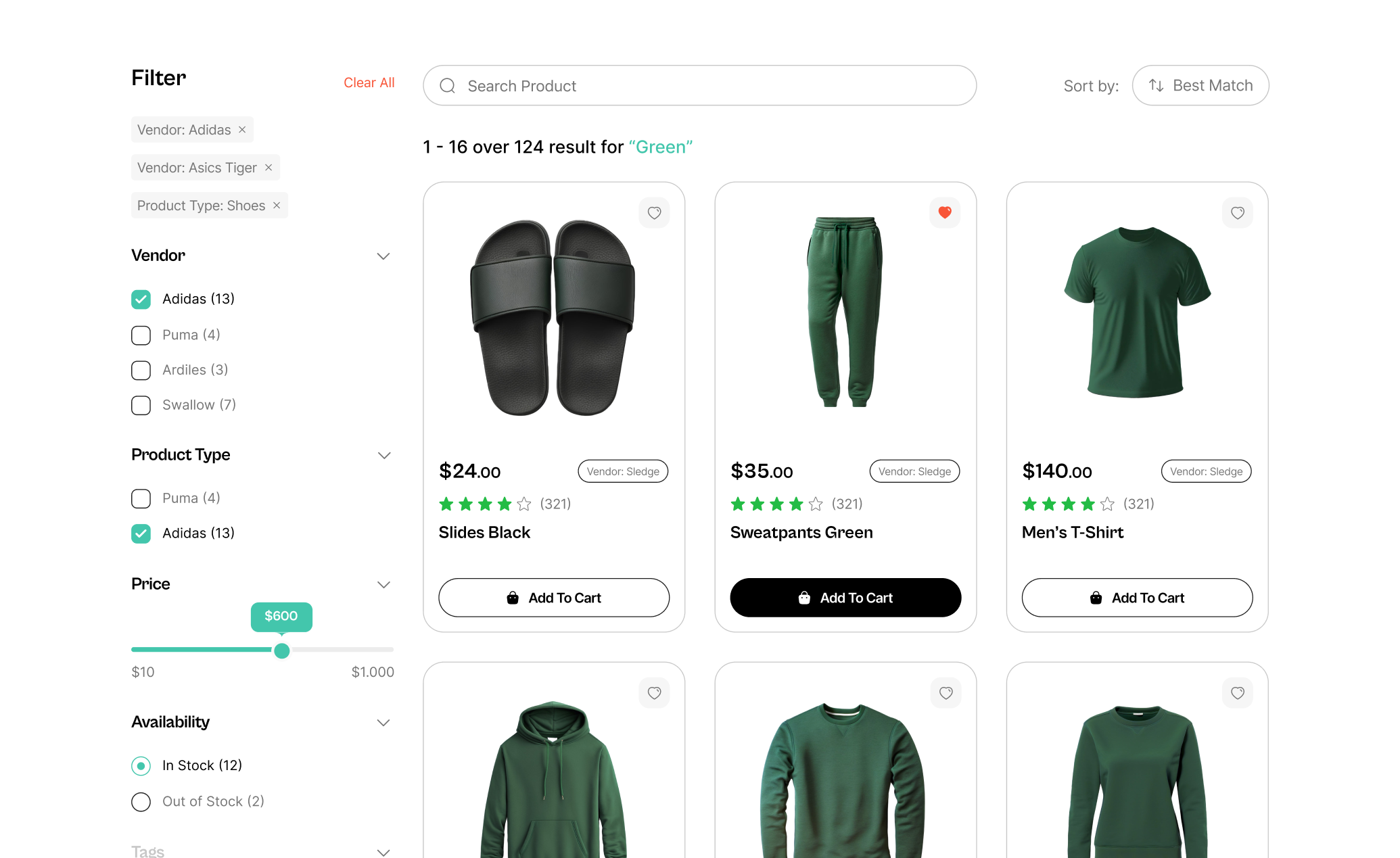
Learn more
Congratulations! Now that the installation is complete, you can use all Sledge's widgets and start integrating to your Hydrogen storefront.