@sledge-app/react-wishlist
You can use our React-built widget by using the @sledge-app/react-wishlist
package.
@sledge-app/react-wishlist
provides several components you can use to integrate it with your store.
Available components :
Badge
Badge is a Wishlist's widget you can use to display your wishlist's total in a small text box or an icon.
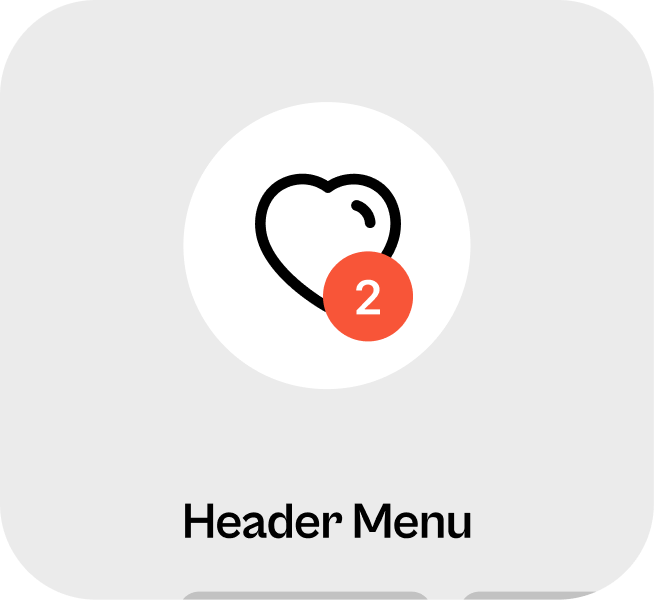
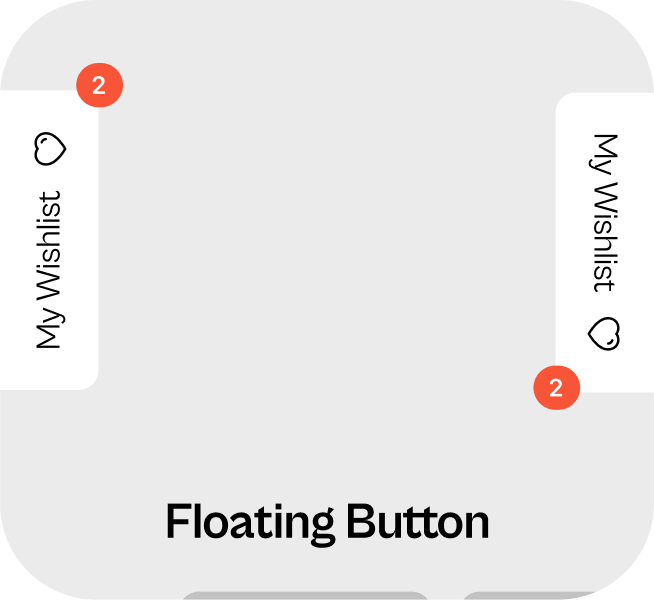
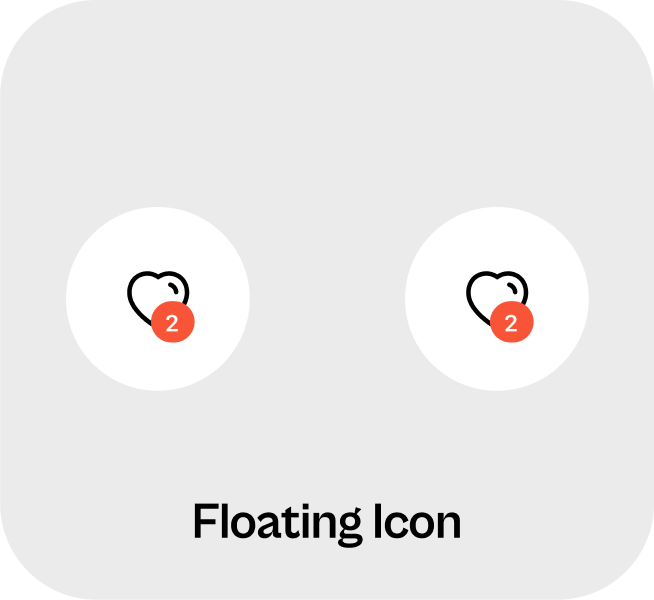
Anatomy
import { Badge } from "@sledge-app/react-wishlist";
import SledgeProductCard from "~/components/SledgeProductCard";
export default function SledgeWishlistBadge() {
return (
<Badge
useProxyUrl={false}
position="left"
useWishlistFlyout={false}
urlWishlistWidget={'/pages/wishlist'}
/>
);
}
API reference
useProxyUrl
Define the url widgets on page that should be displayed (custom or proxy).
- Type:
boolean
- Default:
false
position
Define a widget position for Badge.
- Type:
none
|left
|right
|bottom-left
|bottom-right
- Default:
none
useWishlistFlyout
Display your wishlist items in a sliding panel by activating the Wishlist - Flyout Widget.
- Type:
boolean
- Default:
false
urlWishlistWidget
Fill up the route URL page where your Wishlist widget is located.
https://your-online-store.com/{value}
- Type:
string
Trigger
Trigger is a Wishlist's widget you can use to trigger add or remove a wishlist item in an icon.
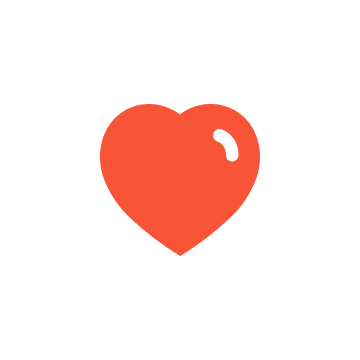
Anatomy
import { Trigger } from "@sledge-app/react-wishlist";
export default function WishlistTrigger() {
return (
<Trigger
params={{
productId: "",
productVariantId: "",
productName: "",
productVendor: "",
productSku: "",
productVariantName: "",
productLink: "",
productImage: "",
productCurrency: "",
productPrice: "",
}}
onAfterAddWishlist={(state) => {
// Your custom function
}}
onAfterRemoveWishlist={(state) => {
// Your custom function
}}
/>
);
}
API reference
params.productId
Fill with your own product id
- Type:
string
params.productVariantId
Fill with your own product variant id
- Type:
string
params.productName
Fill with your own product name
- Type:
string
params.productVendor
Fill with your own product vendor
- Type:
string
params.productSku
Fill with your own product sku
- Type:
string
params.productVariantName
Fill with your own product variant name
- Type:
string
params.productLink
Fill with your own product URL that link to PDP
- Type:
string
params.productImage
Fill with your own product image URL
- Type:
string
params.productCurrency
Fill with your own product currency, eg: IDR
| $
- Type:
string
params.productPrice
Fill with your own product price
- Type:
string
onAfterAddWishlist(state: boolean)
Running using your own custom function
- Type:
function
- Required:
false
onAfterRemoveWishlist(state: boolean)
Running using your own custom function
- Type:
function
- Required:
false
ButtonDetail
Button Detail is a Wishlist's widget you can use to trigger add or remove a wishlist item in a button form. Typically used on product detail page.
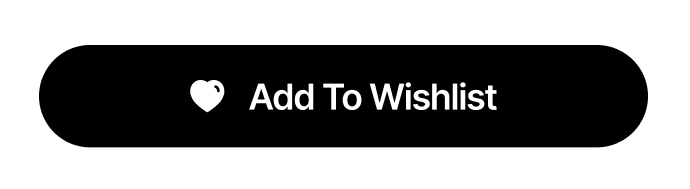
Anatomy
import { ButtonDetail } from "@sledge-app/react-wishlist";
export default function WishlistButtonDetail() {
return (
<ButtonDetail
params={{
productId: "",
productVariantId: "",
productName: "",
productVendor: "",
productSku: "",
productVariantName: "",
productLink: "",
productImage: "",
productCurrency: "",
productPrice: "",
}}
onAfterAddWishlist={(state) => {
// Your custom function
}}
onAfterRemoveWishlist={(state) => {
// Your custom function
}}
/>
);
}
API reference
params.productId
Fill with your own product id
- Type:
string
params.productVariantId
Fill with your own product variant id
- Type:
string
params.productName
Fill with your own product name
- Type:
string
params.productVendor
Fill with your own product vendor
- Type:
string
params.productSku
Fill with your own product sku
- Type:
string
params.productVariantName
Fill with your own product variant name
- Type:
string
params.productLink
Fill with your own product URL that link to PDP
- Type:
string
params.productImage
Fill with your own product image URL
- Type:
string
params.productCurrency
Fill with your own product currency, eg: IDR
| $
- Type:
string
params.productPrice
Fill with your own product price
- Type:
string
onAfterAddWishlist(state: boolean)
Running using your own custom function
- Type:
function
- Required:
false
onAfterRemoveWishlist(state: boolean)
Running using your own custom function
- Type:
function
- Required:
false
Widget
You can use Widget to display list data of user's wishlist.
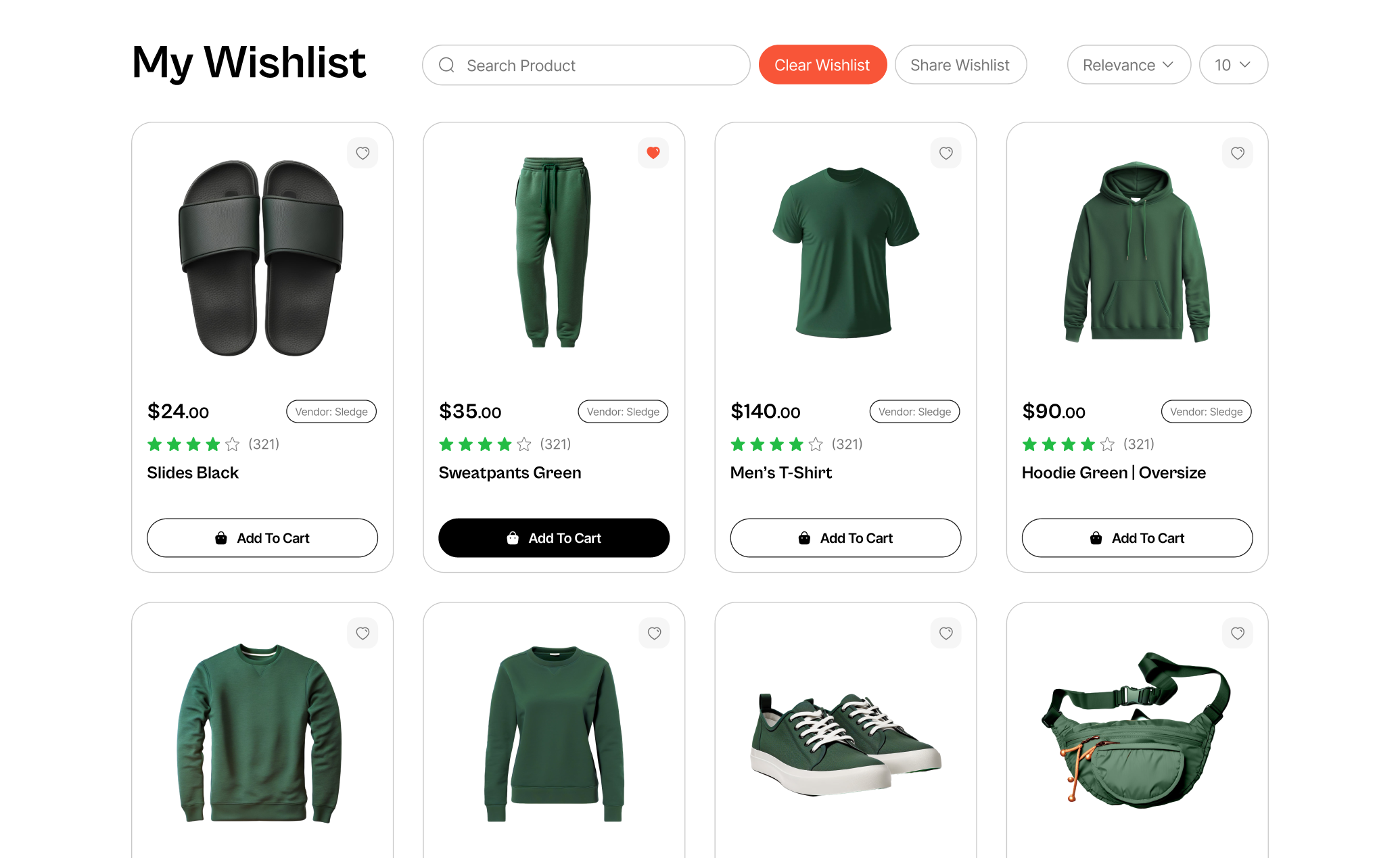
Anatomy
import { Widget, WidgetHeader } from "@sledge-app/react-wishlist";
export default function WishlistWidget() {
return (
<Widget.Root
query={{
shareId: "share",
}}
width="1180px"
limitOptions={[12, 24, 36, 48, 120]}
onAfterAddWishlist={(state) => {
// Your custom function
}}
onAfterRemoveWishlist={(state) => {
// Your custom function
}}
onAfterRenderProduct={(state) => {
// Your custom function
}}
>
<WidgetHeader>
<WidgetHeader.Title text="My Wishlist" />
<WidgetHeader.SearchForm placeholder="Search product" />
<WidgetHeader.ClearTrigger buttonText="Clear Wishlist" />
<WidgetHeader.ShareTrigger buttonText="Share Wishlist" />
<WidgetHeader.Sort />
<WidgetHeader.Limit />
</WidgetHeader>
<Widget.List gridType="large" />
</Widget.Root>
);
}
API reference
<Widget.Root>
query.shareId
Fill up your unique queryParam name for share id.
https://your-online-store.com/pages/wishlist/?{value}=
- Type:
string
- Default:
'share'
width
Customize max width of element.
- Type:
string
- Default:
'1180px'
limitOptions
Customize limit options in widget.
- Type:
string[] | number[]
- Default:
[12, 24, 36, 48, 120]
onAfterAddWishlist(state: boolean)
Running using your own custom function
- Type:
function
- Required:
false
onAfterRemoveWishlist(state: boolean)
Running using your own custom function
- Type:
function
- Required:
false
onAfterRenderProduct(state: boolean)
Running using your own custom function
- Type:
function
- Required:
false
<WidgetHeader.Title>
text
Customize header title in widget
- Type:
string
- Default:
'My Wishlist'
<WidgetHeader.SearchForm>
placeholder
Customize placeholder search form in widget
- Type:
string
- Default:
'Search product...'
<WidgetHeader.ClearTrigger>
buttonText
Customize clear button title in widget
- Type:
string
- Default:
'Clear Wishlist'
<WidgetHeader.ShareTrigger>
buttonText
Customize share button title in widget
- Type:
string
- Default:
'Share Wishlist'
<Widget.Limit>
gridType
Customize grid size product card in widget
- Type:
'small' | 'medium' | 'large'
- Default:
'large'
Learn more
Congratulations! Now that you've learned the @sledge-app/react-wishlist
API references, you can start integrating Sledge to your store. Useful resources about integrating Sledge :